Binary Trees in Swift
Binary trees are a cornerstone of efficient data organisation and management in computer science. Mastering binary trees in Swift not only enhances a developer's toolkit but also provides a deep understanding of how dynamic data structures can be leveraged to optimize algorithms and applications. This article delves into the world of binary trees, from their basic concepts to advanced implementations, and presents practical guidance for Swift developers to excel in this domain.
Key Takeaways
- Understanding the structure and properties of binary trees is foundational for implementing more complex data structures and algorithms in Swift.
- Binary search trees (BSTs) enhance Swift data structures by providing efficient search, insertion, and deletion operations, with performance relying on tree balance.
- Advanced binary tree structures like AVL, Red Black, B Trees, and B+ Trees address the need for self-balancing to maintain optimized performance in Swift applications.
- Algorithmic strategies for binary trees involve searching, sorting, and complexity analysis, which are crucial for real-world applications and performance tuning.
- Identifying common pitfalls and adopting best practices in Swift binary tree implementations ensure maintainable, scalable, and optimized code.
Fundamentals of Binary Trees in Swift
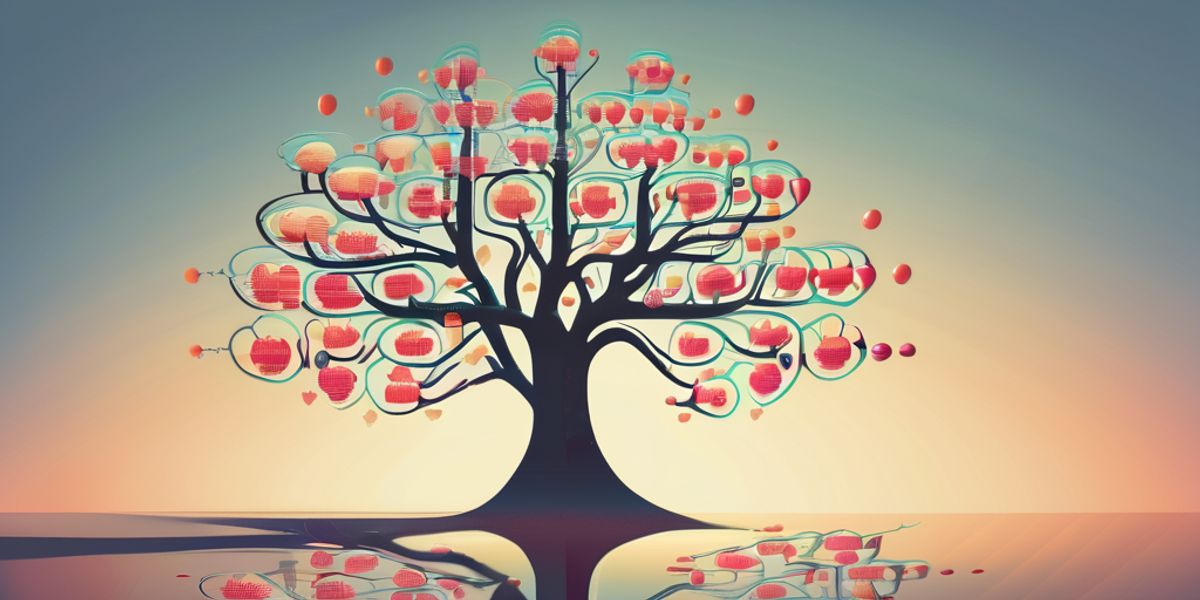
Defining Binary Trees and Their Properties
A binary tree is a hierarchical data structure where each node has at most two children, commonly referred to as the left and right child. In Swift, binary trees can be elegantly represented using recursive enumerations, which align with the language's emphasis on type safety and immutability.
Binary trees are versatile and serve as the foundation for more complex tree structures like binary search trees, AVL trees, and red-black trees.
The properties of binary trees are crucial for understanding their behavior and applications. Here is a list of some fundamental properties:
- Each node contains a value and pointers to its children.
- The topmost node is called the root.
- A node with no children is termed a leaf.
- The height of a tree is the length of the longest path from the root to a leaf.
Binary trees are widely used in various fields, from simple data storage to complex algorithms. Their efficiency and adaptability make them an invaluable tool in computer science.
Implementing a Basic Binary Tree in Swift
In Swift, a basic binary tree can be implemented using a class or struct to represent each node. Each node typically contains a value, along with optional references to its left and right children. The key to a successful implementation is understanding the recursive nature of binary trees, where each child node can be considered a subtree.
To create a binary tree from an array, one can utilize a priority queue to ensure that the tree maintains its properties during construction. The steps involved in this process include initializing the priority queue, inserting elements, and linking nodes appropriately to form the tree structure.
When implementing a binary tree, it's crucial to handle edge cases, such as inserting into an empty tree or removing nodes with one or no children, with care to maintain the integrity of the tree.
Below is a list of operations that are fundamental to managing a binary tree in Swift:
- Top and Peek: Retrieve the highest priority element without removing it.
- Insert: Add a new element to the tree, preserving the binary tree structure.
- Remove: Delete an element from the tree, adjusting the structure as necessary.
- Update Priority: Modify the priority of an element, reordering the tree if required.
These operations form the basis of more complex tree manipulations and are essential for anyone looking to master binary trees in Swift.
Traversal Techniques: In-Order, Pre-Order, and Post-Order
Traversal of a binary tree is a fundamental operation that allows us to visit all the nodes in a structured manner. In-order traversal ensures that we access the nodes in a non-decreasing order, which is particularly useful for binary search trees. Pre-order traversal visits the root before its subtrees, making it suitable for creating a copy of the tree. Post-order traversal, on the other hand, visits the root after its subtrees, which is helpful for deleting the tree.
Traversal methods are not only a means to visit nodes but also a foundation for more complex operations such as searching and sorting within tree structures.
The choice of traversal technique can significantly affect the performance and outcomes of tree-based algorithms. Here is a comparison of the traversal methods:
- In-Order: Left subtree, Node, Right subtree
- Pre-Order: Node, Left subtree, Right subtree
- Post-Order: Left subtree, Right subtree, Node
Understanding the nuances of each method is crucial for effective algorithm design and implementation in Swift.
Binary Search Trees: Enhancing Swift Data Structures
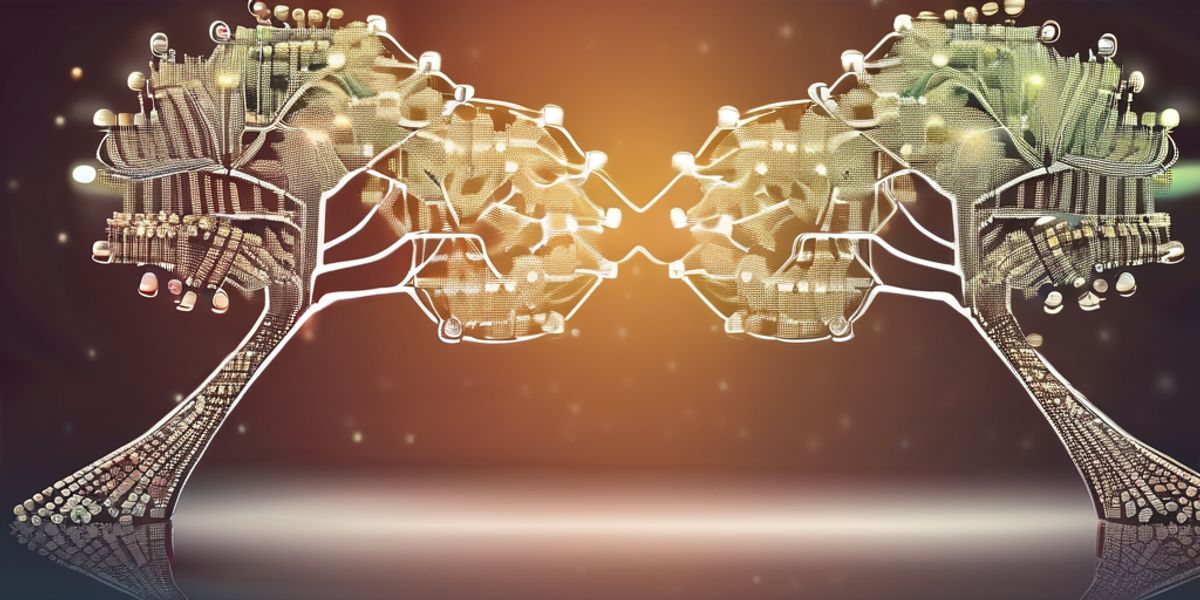
Concept and Characteristics of Binary Search Trees
A Binary Search Tree (BST) is a pivotal data structure in computer science, renowned for its efficiency in managing dynamic data sets. Each node in a BST contains a unique key and references to its left and right children, ensuring that for any given node, all keys in the left subtree are less than the node's key, and all keys in the right subtree are greater. This property is the cornerstone of the BST's ability to expedite search operations.
The characteristics of a BST facilitate swift searches and dynamic data organization, making it a preferred choice for various applications. A BST allows for efficient data retrieval, insertion, and deletion operations, each with an average time complexity of O(log n) in a balanced tree. However, the performance of a BST is heavily dependent on its structure; an imbalanced tree can degrade to O(n) complexity for these operations.
The optimal performance of binary search trees is achieved through height-balancing mechanisms, such as those employed by AVL trees. Ensuring that the tree remains balanced after each insertion or deletion is crucial for maintaining the efficiency of the BST.
In summary, the binary search tree is a versatile and efficient data structure that is widely used in programming and computer science. Its ability to adapt to dynamic data sets while maintaining fast search times is a testament to its robust design and implementation.
Swift Implementation of Insertion and Deletion Operations
Implementing insertion and deletion operations in a binary search tree is crucial for maintaining its efficient search capabilities. In Swift, these operations must adhere to the tree's properties to ensure data remains organized and accessible.
For insertion, the process begins by comparing the new value with the root node. If the value is less, the algorithm moves to the left child; if greater, to the right child. This traversal continues recursively until a suitable position is found. Upon finding the correct location, the new node is inserted maintaining the binary search tree's order.
Deletion is slightly more complex, as it may require reorganizing the tree. There are three cases to consider: deleting a leaf node, a node with one child, and a node with two children. Each scenario requires a different approach to preserve the binary search tree structure.
The binary search tree structure enables quick and efficient search, insertion, and deletion operations, ensuring optimal performance.
Understanding and implementing these operations correctly is fundamental for any developer working with binary trees in Swift. Mastery of these techniques contributes to the development of robust and performant data structures.
Balancing Binary Search Trees for Optimal Performance
Achieving optimal performance in binary search trees (BSTs) is crucial for efficient data management and retrieval. Balancing a BST is a key strategy to ensure that the tree remains efficient for operations such as search, insert, and delete. A balanced BST has a minimized height, which directly impacts the complexity of tree operations.
The balance of a binary search tree is often maintained through various algorithms and structures, such as AVL trees and red-black trees. These self-balancing trees adjust their structure after every insertion or deletion to maintain a balanced state.
To illustrate the importance of tree balance, consider the following table showing the average and worst-case complexities for balanced and unbalanced BSTs:
Operation | Balanced BST Complexity | Unbalanced BST Complexity |
---|---|---|
Search | O(log n) | O(n) |
Insert | O(log n) | O(n) |
Delete | O(log n) | O(n) |
Maintaining a balanced state is not just about performance; it also affects the predictability and reliability of the tree's behavior in various applications. By implementing self-balancing mechanisms, developers can ensure that their binary search trees are robust and efficient, regardless of the number of nodes or the sequence of operations performed.
Advanced Binary Tree Structures and Their Swift Implementations
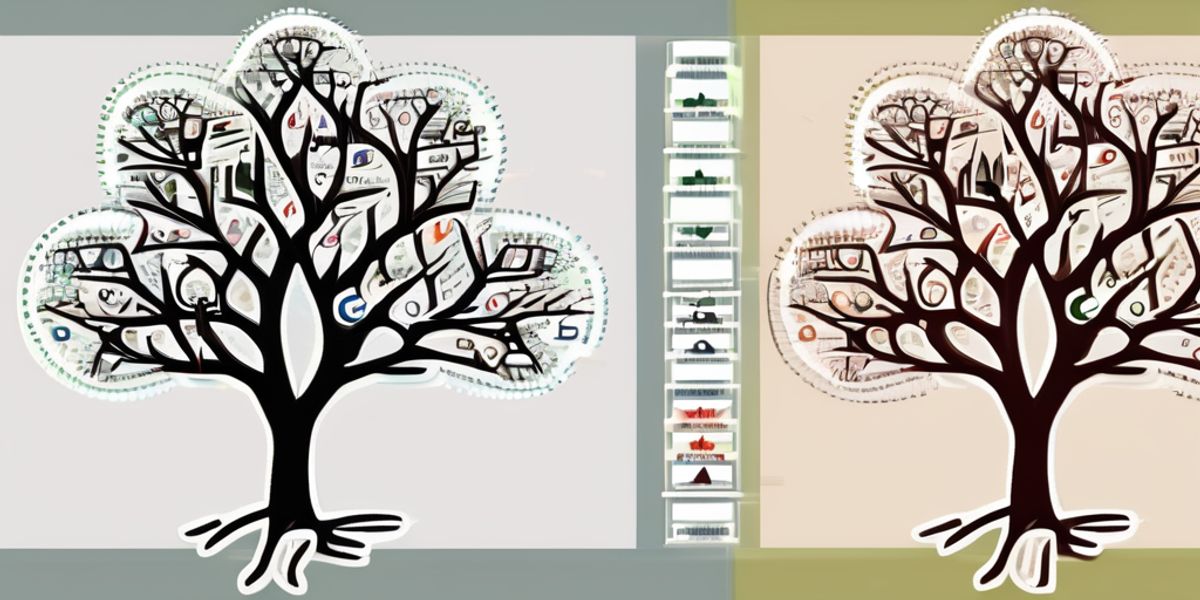
Understanding AVL Trees and Self-Balancing Mechanisms
AVL Trees are a pivotal concept in the realm of data structures, particularly within the context of binary search trees. An AVL Tree is a self-balancing binary search tree, ensuring that the height difference between every node's left and right subtrees is at most one. This characteristic is crucial for maintaining the tree's overall balance, thereby guaranteeing logarithmic time complexity for operations such as insertion, deletion, and lookup.
The self-balancing mechanism of AVL trees is governed by a set of rotations—single or double—that are performed during insertions and deletions. These rotations are pivotal in preserving the tree's balance factor, which is the key to its performance. The balance factor of a node is defined as the height difference between its left and right subtrees. The permissible balance factors are -1, 0, and +1.
The balance factor is maintained after every insertion and deletion, which may require adjustments through rotations. This ensures that the tree remains within the predefined balance factor range, thus maintaining its self-balancing property.
Understanding the implementation of these rotations and the conditions under which they are applied is essential for mastering AVL trees. The following list outlines the basic rotations used in AVL trees:
- Right rotation
- Left rotation
- Right-Left rotation
- Left-Right rotation
Each rotation serves to correct imbalances that occur as a result of modifications to the tree. By mastering these rotations, developers can ensure efficient performance of their AVL tree implementations in Swift.
Red Black Trees: Ensuring Balanced Trees in Swift
Red Black Trees are a sophisticated variant of binary search trees that ensure balanced heights, thereby maintaining optimal search performance. Each node in a Red Black Tree is colored either red or black, following specific rules to balance the tree after insertions and deletions. These rules prevent the tree from becoming significantly unbalanced, which is a common issue in standard binary search trees.
The properties that Red Black Trees must satisfy include:
- Every node is either red or black.
- The root is always black.
- Red nodes cannot have red children (no two red nodes can be adjacent).
- Every path from a node to its descendant NULL nodes must contain the same number of black nodes.
Ensuring that these properties are upheld is crucial for the performance of Red Black Trees. Violations of these properties require specific corrective rotations and color changes to restore balance.
Swift implementations of Red Black Trees benefit from the language's strong type system and memory management features. By leveraging Swift's capabilities, developers can create efficient and reliable tree structures that are essential for applications requiring swift binary searches by halving the search space.
B Trees and B+ Trees: Multi-way Search Trees for Database Indexing
B Trees and B+ Trees are advanced data structures that are essential for efficient database indexing and file systems. Unlike binary search trees, B Trees and B+ Trees allow for more than two children per node, which significantly increases their capacity to handle large datasets. B Trees are particularly well-suited for storage systems that require frequent read and write operations, as they reduce the need for disk I/O by keeping the tree height low.
In the context of Swift, implementing B Trees involves understanding the principles of node splitting and merging. Each node in a B Tree contains a number of keys and child pointers, which must be managed carefully to maintain the tree's sorted order and balance. B+ Trees extend the concept by linking leaf nodes, facilitating efficient range queries and sequential access patterns.
The design of B Trees and B+ Trees is aimed at minimizing the number of disk accesses. Their self-balancing nature ensures that operations such as search, insert, and delete can be performed with logarithmic time complexity, making them indispensable for database systems.
When considering the use of B Trees and B+ Trees in Swift applications, it's important to recognize the trade-offs between complexity and performance. While these structures are more complex than simpler trees, the benefits they provide in terms of scalability and efficiency are substantial, especially in applications dealing with large amounts of data.
Algorithmic Strategies for Binary Trees
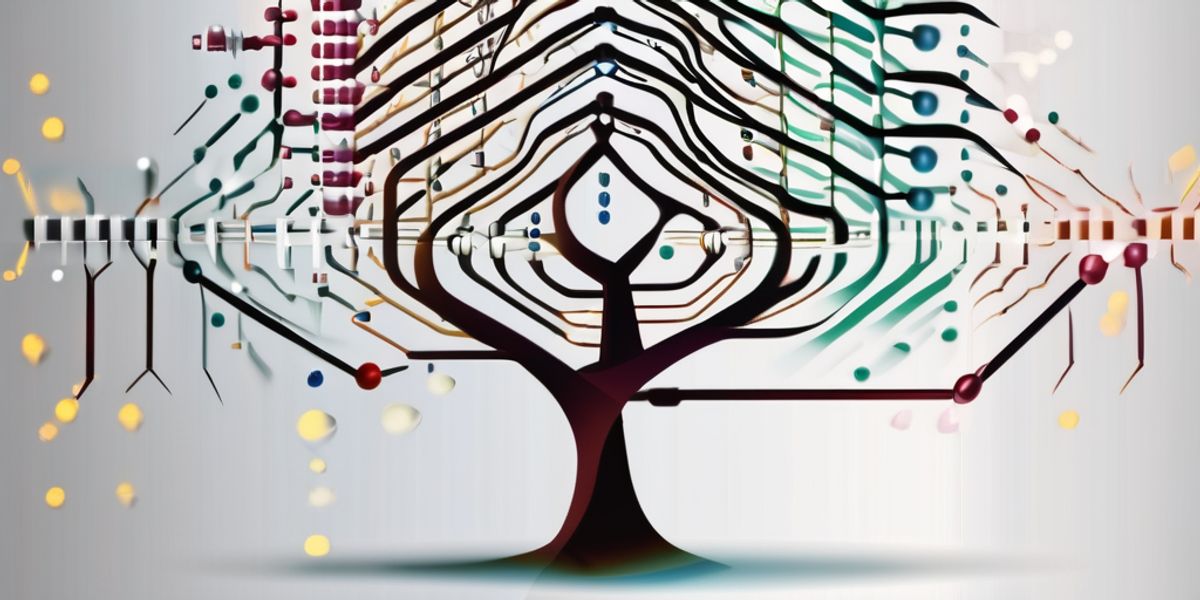
Binary Tree Algorithms: Searching, Sorting, and More
Binary trees are a pivotal data structure in Swift, and mastering their associated algorithms is crucial for efficient problem-solving. Searching algorithms are fundamental in binary trees, with binary search being a prime example. It's essential to note that binary search requires your data to be sorted by the key you seek. Sorting algorithms, on the other hand, are diverse and tailored to different scenarios. Common sorting algorithms include:
- Merge Sort
- Quick Sort
- Heap Sort
- Counting Sort
- Radix Sort
- Bucket Sort
Each algorithm has its own strengths and is chosen based on the specific requirements of the data and the application.
When implementing binary tree algorithms in Swift, it's important to consider the time and space complexity of each algorithm to ensure optimal performance.
In addition to searching and sorting, binary trees in Swift can be utilized for more complex operations such as dynamic programming, backtracking, and graph algorithms. These advanced techniques allow for a more nuanced approach to data manipulation and retrieval, paving the way for sophisticated software solutions.
Complexity Analysis for Binary Tree Operations
Understanding the time and space complexity of binary tree operations is crucial for optimizing performance. The efficiency of these operations is often determined by the height of the tree. For instance, in a balanced binary tree, operations such as insertion, deletion, and search can be performed in O(log n) time, where 'n' is the number of nodes. This is due to the tree's structure, which allows for operations to be executed by traversing only a fraction of the total nodes.
The logarithmic nature of binary search time complexity ensures swift access to elements by halving the search space in each iteration.
However, in the worst case, such as when a binary tree becomes skewed, these operations degrade to O(n) time complexity. It is therefore important to maintain the tree's balance to ensure optimal performance. Below is a table summarizing the average and worst-case time complexities for various binary tree operations:
Operation | Average Case | Worst Case |
---|---|---|
Insertion | O(log n) | O(n) |
Deletion | O(log n) | O(n) |
Search | O(log n) | O(n) |
Traversal | O(n) | O(n) |
Space complexity is another important aspect, with most binary tree operations requiring O(n) space due to the storage of 'n' nodes. However, the auxiliary space for recursive operations may also need to be considered, especially in the context of algorithm implementation in Swift.
Case Studies: Real-World Applications of Binary Trees
Binary trees are a cornerstone of efficient data management in computer science. They are employed across various domains to structure data in a manner that allows for rapid searching, insertion, and deletion operations. Binary trees are particularly prevalent in database systems and file management, where they underpin the indexing mechanisms that facilitate quick data retrieval.
In the realm of education, binary trees are a staple topic. They serve as a fundamental concept for students grappling with data structure homework, often featuring in assignments that require the implementation of various tree types, such as AVL, Red Black, and B+ trees. These exercises not only reinforce theoretical knowledge but also hone practical coding skills.
Binary trees exemplify the synergy between theoretical computer science and practical application, demonstrating their versatility and indispensability in both academic and professional settings.
The following table outlines some common binary tree types and their typical applications:
Binary Tree Type | Typical Application |
---|---|
Generic Tree | Hierarchical Data |
Binary Tree | Basic Data Structure |
Binary Search Tree | Sorted Data Storage |
AVL Tree | Self-Balancing Search Trees |
B Tree | Database Indexing |
B+ Tree | Database Indexing with Sequences |
Red Black Tree | Balanced Search Trees |
Understanding the practical applications of binary trees is crucial for developers and computer scientists alike, as it informs the selection of the appropriate data structure for a given problem.
Common Pitfalls and Best Practices in Swift Binary Tree Implementations
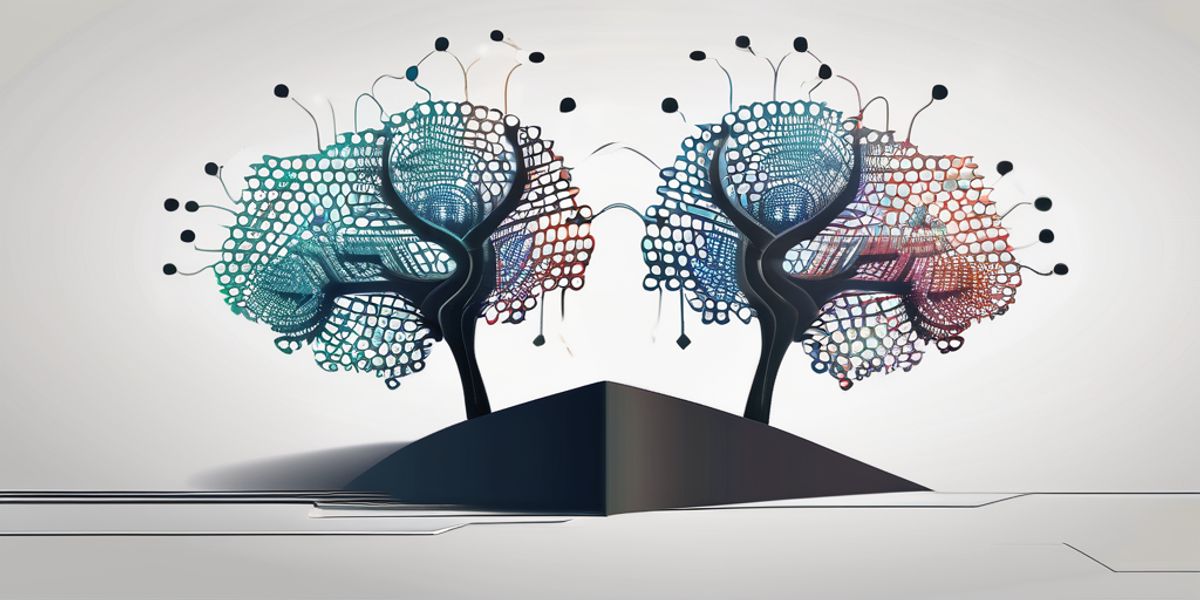
Debugging Common Issues in Binary Tree Code
When working with binary trees in Swift, developers may encounter a range of common issues that can affect the performance and correctness of their code. Identifying and resolving these issues is crucial for ensuring the integrity of the binary tree's structure and its associated operations.
One frequent problem is the improper handling of edge cases, such as inserting or deleting nodes in an empty tree or at the boundaries of the tree. This can lead to incorrect tree states or even crashes. To address this, developers should rigorously test their tree operations with a variety of scenarios, including edge cases.
Another issue is memory leaks, which occur when nodes are removed from the tree without properly deallocating the associated memory. Swift's automatic reference counting (ARC) helps manage memory, but developers must still ensure that all references to nodes are cleared to prevent leaks.
In the context of binary trees, a common pitfall is neglecting the importance of maintaining the tree's balance. An unbalanced tree can degrade to a linked list in the worst case, resulting in O(n) complexity for operations that could be O(log n) with proper balancing.
Below is a list of steps to follow when debugging binary tree code:
- Verify the base structure of the tree after each operation.
- Check for correct implementation of tree traversal methods.
- Use breakpoints and step-through debugging to observe the state of the tree at various stages.
- Implement unit tests covering a wide range of inputs, including boundary conditions.
- Profile the application to identify memory issues or performance bottlenecks.
By systematically addressing these common issues, developers can enhance the reliability and efficiency of their binary tree implementations in Swift.
Performance Optimization Tips for Swift Trees
Optimizing the performance of binary trees in Swift involves a strategic approach to both algorithm design and data structure selection. Benchmarking changes is crucial; by measuring performance before and after optimizations, developers can quantify the impact of their improvements. For instance, profiling might reveal that certain tree operations are bottlenecks, leading to targeted enhancements.
- Identify slow functions and optimize critical paths.
- Use hash maps for fast lookups when dealing with large datasets.
- Employ arrays for constant-time access, and consider trees for efficient searching and sorting.
When optimizing, it's essential to consider the trade-offs between time complexity and memory usage. A well-chosen data structure can significantly reduce the time complexity of operations without excessively increasing memory overhead.
Finally, implementing efficient algorithms and data structures tailored to the application's needs can lead to substantial performance gains. For example, using a balanced tree structure like an AVL or Red-Black tree can improve search times in comparison to an unbalanced binary tree.
Writing Maintainable and Scalable Binary Tree Code in Swift
Writing maintainable and scalable code for binary trees in Swift is crucial for the longevity and efficiency of your applications. Proper structuring and adherence to best practices are key to achieving this goal. Below are some guidelines to follow:
- Use consistent naming conventions for classes, methods, and variables.
- Implement thorough error handling and input validation.
- Apply the principles of object-oriented programming to encapsulate tree functionality.
- Write unit tests for each tree operation to ensure code reliability.
By focusing on clean code and modular design, developers can create binary tree implementations that are both robust and adaptable to changing requirements.
Remember that the complexity of binary tree operations can impact the performance of your application. Here is a quick reference for the average time complexities:
Operation | Average Case Complexity |
---|---|
Insertion | O(log n) |
Deletion | O(log n) |
Search | O(log n) |
Traversal | O(n) |
Maintaining a balance between readability and efficiency is essential. Refactor your code regularly to improve its structure without compromising on performance.
Conclusion
In this comprehensive exploration of binary trees in Swift, we have journeyed from the foundational concepts to the more advanced intricacies of tree-based data structures. We delved into the versatile applications of binary trees, from organizing data efficiently to optimizing search operations. By implementing various types of binary trees, such as AVL and Red-Black Trees, we have seen how Swift's robust features can be leveraged to achieve performance and reliability in our data structures. As we conclude, it is evident that mastering binary trees is not only about understanding their theoretical underpinnings but also about practical implementation and optimization. The knowledge and skills acquired here are crucial for any Swift developer looking to excel in the realm of data structures and algorithms. May this article serve as a stepping stone for further exploration and mastery of computer science fundamentals within the Swift programming landscape.
Frequently Asked Questions
What is a Binary Tree in Swift and how is it used?
In Swift, a Binary Tree is a hierarchical data structure where each node has at most two children, referred to as the left child and the right child. It is used to represent data with a branching structure, allowing for efficient insertion, deletion, and searching operations.
How do you implement a Binary Search Tree in Swift?
A Binary Search Tree (BST) in Swift is implemented by creating a Node class with key, left child, and right child properties. The BST maintains the property that for any given node, all elements in the left subtree are less than the node's key, and all elements in the right subtree are greater.
What are the different ways to traverse a Binary Tree?
There are three primary ways to traverse a Binary Tree: In-Order (left, root, right), Pre-Order (root, left, right), and Post-Order (left, right, root). Each traversal method serves different purposes and yields the nodes in a specific order.
Why is balancing important in Binary Search Trees?
Balancing is crucial in Binary Search Trees to maintain a lower height, which ensures operations like search, insert, and delete can be performed in O(log n) time complexity. Without balancing, the height can become linear, degrading performance to O(n).
How do advanced tree structures like AVL and Red Black Trees differ from basic Binary Trees?
Advanced tree structures like AVL and Red Black Trees include mechanisms to automatically balance themselves after insertions and deletions. This ensures that the trees remain approximately balanced at all times, providing better performance guarantees for dynamic sets of data.
What are some common pitfalls when working with Binary Trees in Swift?
Common pitfalls include not properly handling edge cases, such as inserting into an empty tree or deleting a node with two children, and neglecting to rebalance the tree after operations that modify its structure. These can lead to incorrect results or suboptimal performance.
Sign up here with your email
ConversionConversion EmoticonEmoticon