Swiftly Sorted: Mastering Data Structures and Algorithms for iOS Development
Swift, the powerful programming language tailored for iOS development, has evolved significantly since its introduction. Understanding and mastering data structures and algorithms in Swift is crucial for developers looking to optimize performance, maintainability, and scalability in their iOS applications. This article delves into the intricacies of Swift's data handling capabilities, explores algorithmic strategies for enhanced app performance, and discusses the implementation of advanced data structures. We'll also look at the latest Swift features for algorithm optimization and navigate the critical aspects of data security and privacy in iOS development.
Key Takeaways
- Swift's evolution has brought sophisticated data handling capabilities, including generics and type-safety features, which are essential for robust iOS app development.
- Implementing efficient algorithms in Swift, such as linked lists and enums, significantly improves app performance and memory management.
- Advanced data structures, like parsing Mach-O files, demonstrate Swift's versatility in handling complex data representation scenarios.
- Optimization techniques, including whole module optimization and dynamic runtime performance, are key to achieving high-performing Swift applications.
- Data security and privacy are paramount in Swift applications, with iOS updates introducing changes that developers must adapt to ensure user data protection.
Understanding Swift's Evolution in Data Handling
Swift 2 Generic Support for @objc Protocols
With the advent of Swift 2, developers were introduced to enhanced generic support for @objc
protocols, marking a significant evolution in data handling. This feature bridged the gap between Swift's type safety and the dynamic nature of Objective-C.
Swift's strong typing system was further empowered by allowing generics to conform to @objc
protocols, which was not possible in earlier versions. This opened up new possibilities for developers to write more expressive and flexible code while maintaining performance.
The introduction of generic support for @objc protocols in Swift 2 was a pivotal moment for iOS developers, as it provided a pathway to leverage the full potential of Swift's modern features in conjunction with existing Objective-C APIs.
Here is a brief overview of the benefits this feature brought to Swift development:
- Enhanced code reusability and maintainability.
- Improved interoperability with Objective-C APIs.
- The ability to define protocols that can be adopted by classes, structs, and enums.
This feature was a testament to Swift's commitment to evolving alongside developers' needs, ensuring that the language remains both powerful and accessible.
Efficiently Mutating Nested Swift Data Structures
Swift's ability to handle complex data structures has evolved significantly, allowing developers to efficiently mutate nested structures with ease. This capability is crucial when dealing with hierarchical data, such as JSON responses from web services or complex user interface states.
- Value semantics in Swift ensure that when you copy a structure, you get a distinct instance, which is essential for predictable behavior during mutations.
- Copy-on-write optimization helps maintain performance by delaying the copying of data until it is actually modified.
- Using key paths and functional programming techniques, Swift developers can update deeply nested structures in an expressive and safe manner.
Swift's standard library and third-party packages offer a variety of tools to simplify these tasks. For instance, the swift-collections package aims to provide a set of data structures that should be part of every Swift engineer's toolbox.
By leveraging these features and tools, Swift developers can write code that is not only more maintainable but also performs better when dealing with complex data mutations.
Type-Safe User Defaults in Swift
Swift's UserDefaults
system provides a flexible way to store lightweight data. However, it's traditionally been prone to errors due to its untyped nature. Type-safety can be achieved by wrapping UserDefaults
in a custom structure that leverages Swift's strong typing. This ensures that the data we save and load is correctly typed, reducing runtime errors and improving code quality.
For instance, consider the following example where we define a Settings
struct that encapsulates user preferences:
struct Settings {
static var username: String {
get { return UserDefaults.standard.string(forKey: "username") ?? "" }
set { UserDefaults.standard.set(newValue, forKey: "username") }
}
// Add more properties here
}
By using this pattern, we can fix this by making the Prospects
initializer able to load data from UserDefaults
, then write it back when the data changes. This time our data is stored in a type-safe manner, minimizing the risk of bugs and simplifying the debugging process.
Mastering Algorithms in Swift for Enhanced Performance
Linked Lists, Enums, Value Types and Identity
Swift's approach to data structures is exemplified in its implementation of linked lists. Unlike traditional object-oriented languages where linked lists are typically reference types, Swift encourages the use of value types for such constructs. This shift enhances safety and performance, as value types are copied rather than referenced, preventing unintended mutations and simplifying memory management.
Enums in Swift are not just simple lists of constants but can be associated with values of any given type, making them powerful tools for creating expressive and type-safe data structures. When combined with linked lists, enums can represent nodes with associated data in a strongly-typed manner, ensuring that errors are caught at compile-time rather than at runtime.
Identity is another crucial aspect when dealing with data structures in Swift. Since value types do not have an inherent identity, understanding when to use reference types, which do carry an identity, is key for certain algorithms where the identity of an object is important, such as in caching mechanisms or when tracking unique instances.
Swift's design encourages developers to think more deeply about the data they are manipulating, promoting safer and more predictable code patterns.
Swift.Codable for Robust Data Encoding and Decoding
Swift's Codable
protocol is a cornerstone in modern iOS development, allowing for seamless conversion between JSON and Swift objects. By conforming to Codable
, developers can easily encode and decode data, streamlining the process of working with APIs and data persistence.
- Encode: Convert Swift objects to JSON or other formats.
- Decode: Reconstruct Swift objects from JSON or other encoded forms.
This protocol not only simplifies code but also enhances safety by ensuring type consistency throughout the process. For example, when mapping Swift objects to JSON, the compiler can catch potential mismatches at compile-time, reducing runtime errors.
By leveraging Codable, developers can focus on the logic and functionality of their apps, rather than the intricacies of data conversion.
Understanding and implementing Codable
effectively can lead to cleaner, more maintainable code. It's a skill that, once mastered, significantly boosts the robustness of data handling in Swift applications.
Patterns to Avoid Massive View Controllers
Massive view controllers are a common pitfall in iOS development, often resulting from an accumulation of responsibilities that should be distributed across multiple classes or structures. Refactoring these 'monoliths' is crucial for maintainability and scalability. To combat this anti-pattern, developers can employ several strategies:
- Utilize design patterns such as MVVM, MVP, or Coordinator to separate concerns.
- Delegate responsibilities to helper classes or services.
- Leverage Swift's extensions to keep view controllers concise.
- Implement protocols to define clear interfaces between components.
Embracing these patterns not only clarifies the architecture but also facilitates unit testing and debugging. By adhering to the Single Responsibility Principle, each component becomes more focused and manageable.
Choosing the right design pattern depends on the specific needs of the app and the team's familiarity with the pattern. It's important to remember that the goal is to create a harmonious balance between simplicity and functionality, avoiding the extremes of both over-engineering and under-structuring.
Leveraging Swift for Advanced Data Structure Implementation
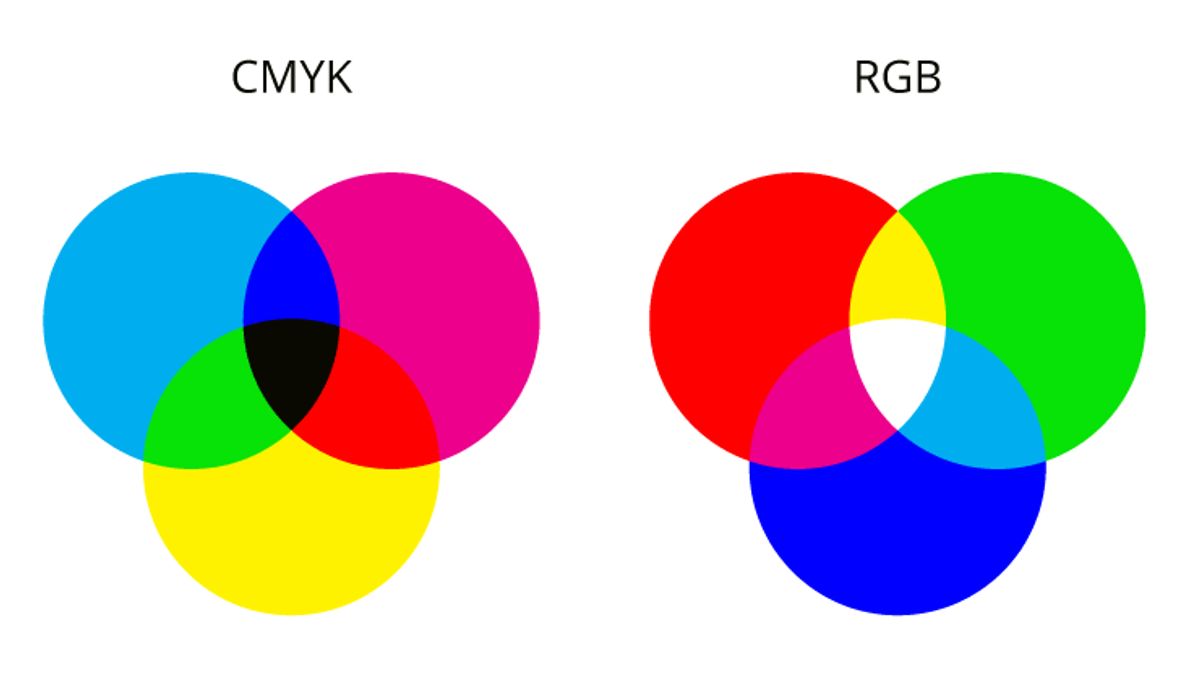
Parsing Mach-O Files and Data Representation
Mach-O files, standing for Mach Object, are a file format for executables, object code, shared libraries, and core dumps in macOS and iOS. Understanding the structure of Mach-O files is crucial for developers, especially when dealing with low-level data representation and optimization. Parsing these files can be intricate, involving multiple steps and considerations.
The process of parsing Mach-O files typically begins with identifying the magic number that signifies the file type, followed by reading the headers that describe the architecture and layout of the file. Developers can then extract and manipulate the data sections and symbols as needed for their applications.
The ability to parse and understand Mach-O files is a valuable skill for any developer working on Apple platforms, providing insights into the compilation process and binary composition.
For example, parsing Mach-O files with Python using Lief involves three initial steps: Importing lief, specifying the Mach-O file to open, and parsing the file as needed. This process is not only relevant for developers but also for security analysts and reverse engineers who need to dissect binary files for various purposes.
The Role of Protocols in Data Structure Design
In Swift, protocols are fundamental to designing flexible and reusable data structures. Protocols define a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality. By conforming to protocols, data structures can guarantee they offer the necessary functionality, which is especially useful when the structures are to be used in a variety of different contexts.
- Protocols encourage decoupling, allowing for more modular code.
- They enable polymorphism, letting developers work with types based on their behavioral promises rather than their specific types.
- Protocols can be extended to provide default implementations, reducing code duplication.
Protocols are not just a tool for abstraction but also a means of ensuring consistency across different parts of an application. They serve as a contract, ensuring that any data structure that conforms to the protocol will behave in a predictable manner, which is crucial for maintaining a clean and understandable codebase.
Weak and Unowned References in Swift
In Swift, managing memory efficiently is crucial, and understanding the difference between weak and unowned references is a key part of this. Weak references allow one to reference an object without creating a strong hold on it, preventing retain cycles that can lead to memory leaks. Unlike weak references, unowned references do not increase the reference count of the object they point to, and they are not optional.
When using unowned references, it's important to ensure that the referenced object will not be deallocated before the unowned reference is used. This is because unowned references do not automatically become nil when the object they reference is deallocated, leading to potential crashes if not handled correctly.
Here's a comparison of weak and unowned references in Swift:
- Weak references are always optional and automatically become nil when the referenced object is deallocated.
- Unowned references are non-optional and must not be accessed after the referenced object has been deallocated.
- Both weak and unowned references do not create strong holds on the referenced object, helping to prevent retain cycles.
Optimizing Data Algorithms with Swift's Latest Features
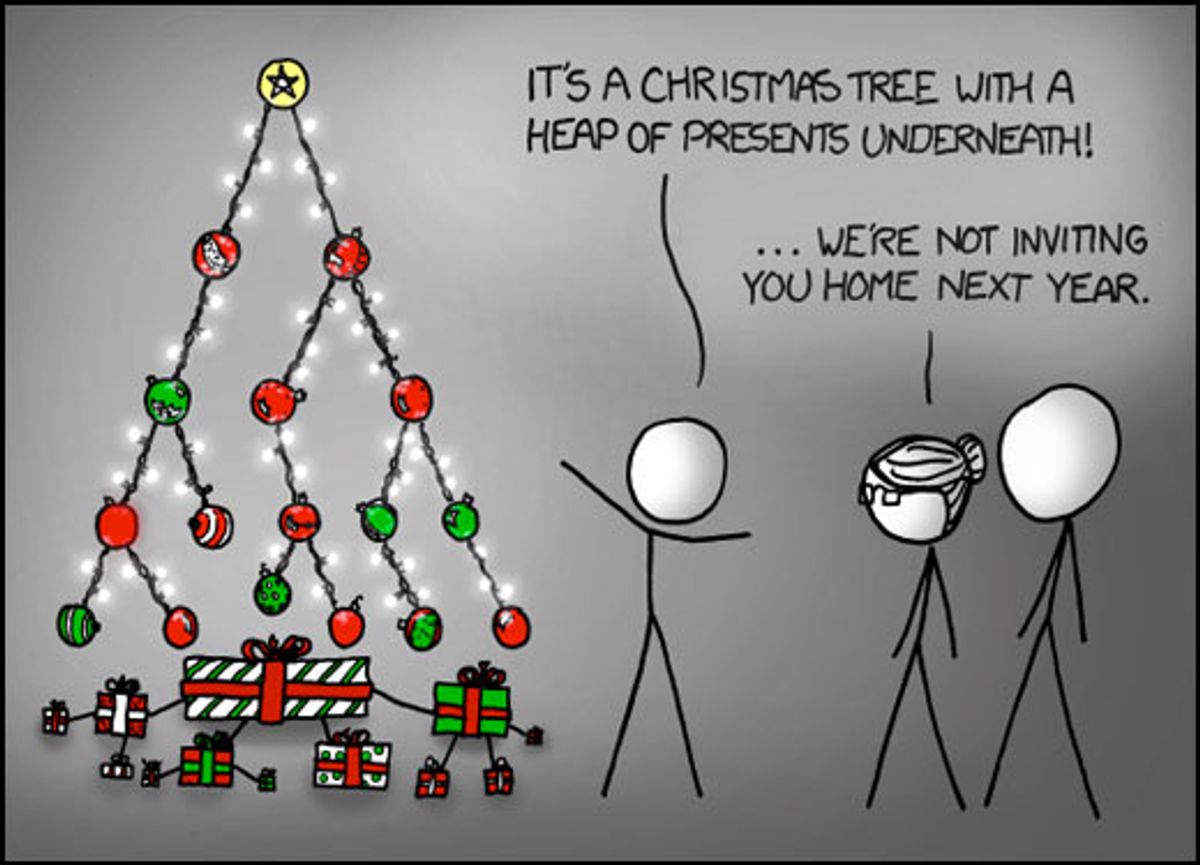
Swift Whole Module Optimization
Swift's Whole Module Optimization (WMO) is a powerful feature that can significantly improve the performance of your applications. WMO allows the Swift compiler to optimize across all the files in a module, leading to faster compile times and more efficient code. When enabled, the compiler has a holistic view of the entire module, which facilitates aggressive optimizations that are not possible when files are compiled individually.
Whole Module Optimization is particularly beneficial for projects with a large codebase, as it can drastically reduce the build time and enhance the runtime performance of the application.
To take advantage of WMO, you can enable it in your project's build settings. Here's a simple list of steps to follow:
- Navigate to your project's build settings in Xcode.
- Search for 'Swift Compiler - Code Generation'.
- Set the 'Optimization Level' to 'Whole Module Optimization'.
Remember, while WMO can offer significant performance gains, it may also increase the memory usage during compilation. It's essential to weigh the benefits against the potential trade-offs for your specific project.
Dynamic Swift and Runtime Performance
Swift's dynamic features have been a topic of interest for developers aiming to optimize runtime performance. Dynamic Swift allows for more flexible and powerful code, which can be particularly useful in scenarios where static typing can be too restrictive.
- Dynamic dispatch is a key feature that enables runtime decisions about which method to call.
- Swift's
@dynamicCallable
and@dynamicMemberLookup
attributes provide dynamic behavior akin to scripting languages. - The use of dynamic features should be balanced with the need for type safety and performance.
Swift's dynamic capabilities, when used judiciously, can lead to more expressive and adaptable codebases. However, developers must be cautious not to overuse these features, as they can potentially lead to harder-to-maintain code and performance bottlenecks.
Strings in Swift 2 and Memory Management
Swift's approach to memory management is a cornerstone of efficient iOS app development. Automatic Reference Counting (ARC) ensures that memory is managed automatically, but developers must still be vigilant to avoid memory leaks and retain cycles. A common challenge arises with the management of strings, as they are often used extensively in apps and can be a source of memory inefficiency if not handled correctly.
In Swift 2, strings are value types and are copied when assigned to a new variable or constant, unless they are mutated. This copy-on-write strategy is optimized for performance, but it requires developers to understand when copies are made to manage memory effectively. To illustrate, consider the following table showing the behavior of strings in different scenarios:
Operation | Copy Behavior |
---|---|
Assignment | No copy if not mutated |
Mutation | Copy on write |
Passing to Function | No copy if not mutated |
By mastering these behaviors, developers can optimize their app's efficiency. It's also crucial to comprehend how reference types like classes interact with value types like strings, especially when closures or capture lists are involved.
Swift's memory management and ARC are essential for preventing reference cycles and memory leaks. Understanding weak and unowned references is key to optimizing your app with best practices.
Navigating Data Security and Privacy in Swift Applications
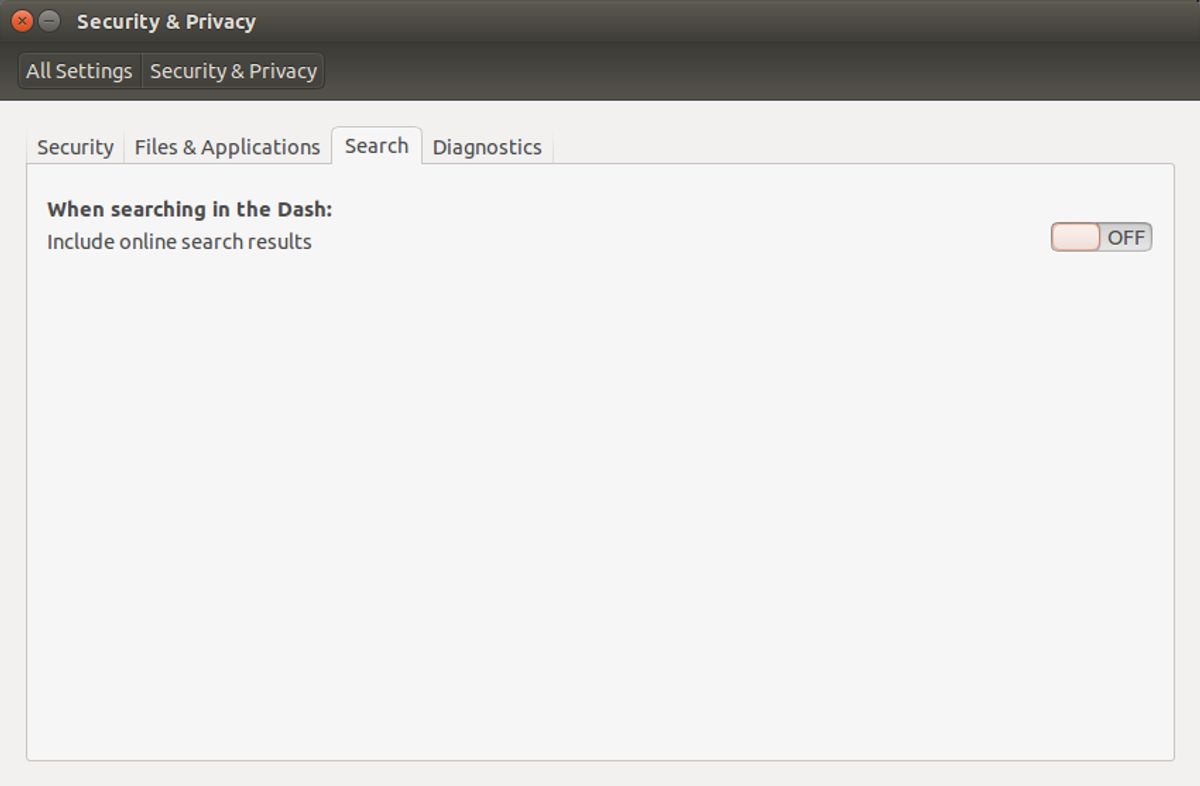
Security and Privacy Changes in iOS 9
With the release of iOS 9, Apple introduced several enhancements to improve user security and privacy. One of the most significant changes was the strengthening of the encryption system, which now extends to more user data than ever before. This includes protection for Notes, Reminders, and other app data when the device is locked with a passcode.
Another key update was the refinement of Location Services. Users gained more control over their privacy with the ability to grant app access to location data only while the app is in use. Additionally, the Night Shift feature, which adjusts screen colors to reduce blue light exposure, was also introduced in this version.
The focus on privacy is evident in the way iOS 9 handles app permissions, requiring apps to request access each time they need to use sensitive components like the microphone or camera.
The table below summarizes some of the privacy-related features that were either introduced or improved in iOS 9:
Feature | Description |
---|---|
Encryption | Enhanced encryption for Notes, Reminders, and app data with passcode |
Location Services | More granular control over app access to location data |
Night Shift | Reduces blue light exposure by adjusting screen colors |
Users are reminded that they can change their mind anytime and update their preferences from Settings > Privacy & Security > Location Services.
Safari Safe Browsing, China, and Privacy
In the realm of data security and privacy, Safari's Safe Browsing feature stands as a significant tool for protecting users from malicious websites. However, the implementation of this feature in China presents unique challenges. The Chinese government employs stringent internet regulations, often referred to as the 'Great Firewall of China', which includes the blocking of thousands of sites deemed inappropriate or politically sensitive.
- Websites and apps blocked in China are a testament to the country's tight control over internet content. This censorship affects not only domestic platforms but also international services that fail to comply with local laws.
The balance between user privacy and regulatory compliance is a delicate one, especially in regions with strict censorship laws.
Apple's approach to privacy has been both praised and criticized. On one hand, the company has made strides in enhancing user privacy with features like App Tracking Transparency. On the other hand, it has faced scrutiny for its compliance with government requests, which sometimes involves the removal of certain apps from the App Store in China.
iOS Default File Associations and Data Protection
With the release of iOS, Apple has tightened the integration between file types and their associated applications, ensuring a more secure and streamlined user experience. Data protection has been elevated, with the system providing robust encryption for files by default. This change is particularly significant for sensitive information that users handle on their devices.
- Default file associations prevent malicious apps from hijacking file types.
- Data protection is enforced using strong encryption algorithms.
- Users have more control over which apps can access their files.
The seamless integration of file associations with data protection mechanisms underscores Apple's commitment to user privacy and security. It not only simplifies the user experience but also fortifies the data against unauthorized access.
Developers must now adhere to stricter guidelines when handling files, which has implications for app design and functionality. The implications of these changes are far-reaching, affecting everything from user privacy to the way developers approach app security.
Conclusion
As we wrap up our exploration of data structures and algorithms in Swift, it's clear that mastering these concepts is crucial for developing efficient and effective iOS applications. Swift's evolution, including its support for generics and protocol-oriented programming, has empowered developers to write safer, more reusable code. The journey through various data structures, from linked lists to trees, and the implementation of algorithms, from sorting to searching, has equipped us with the tools to tackle complex problems with confidence. Whether you're refactoring legacy code or building new features, the knowledge of data structures and algorithms will serve as a foundation for writing high-quality Swift code. Remember, the beauty of Swift lies in its blend of simplicity and power—embrace it, and you'll unlock the full potential of iOS development.
Frequently Asked Questions
What improvements did Swift 2 introduce for handling generics with @objc protocols?
Swift 2 introduced enhanced support for generics that allowed for more robust type checking and interoperability with Objective-C, particularly with the use of @objc protocols. This improvement enabled developers to write more flexible and type-safe code when interacting with Objective-C APIs.
How can nested data structures be mutated efficiently in Swift?
Efficient mutation of nested data structures in Swift can be achieved by utilizing value types like structs, which allow for copy-on-write semantics, and by leveraging functional programming techniques to transform data without side effects.
What is Swift.Codable and how does it improve data encoding and decoding?
Swift.Codable is a protocol-oriented programming feature introduced in Swift 4 that simplifies the process of encoding and decoding data types to and from different formats such as JSON and XML. It provides a more robust and less error-prone approach to handling data serialization.
What are some patterns to avoid massive view controllers in Swift applications?
To avoid massive view controllers in Swift, developers can use design patterns such as MVVM, Coordinator, and MVC-N, as well as techniques like dependency injection, protocol-oriented programming, and breaking down view controllers into smaller, more manageable components.
How does Swift ensure data security and privacy in applications?
Swift ensures data security and privacy through various mechanisms such as enforcing strong typing, using access control to protect data, leveraging Apple's security frameworks like Keychain and Data Protection, and conforming to privacy guidelines set by Apple for iOS applications.
What are the benefits of Swift's Whole Module Optimization?
Swift's Whole Module Optimization (WMO) is a build setting that compiles the entire module at once, allowing the compiler to optimize across all files within the module. This results in improved performance, faster execution times, and reduced binary sizes.
Sign up here with your email
ConversionConversion EmoticonEmoticon